앱들을 사용하다 보면 스크롤할 때나 상단에 검색 툴바, 하단의 바가 사라질 때가 있고 나타날 때가 있다.
이러한 효과를 사용해보기 위함도 있고 코드본을 가지고 있기 위해 구현해 보았다.
CoordinatorLayout
먼저 숨김처리 효과를 구현하기 위해 CoordinatorLayout을 사용해야 한다.
CoordinatorLayout의 Behavior를 통해 자식 뷰들 간에 상호작용을 제공한다.
이 Layout을 이용하면 자식 뷰들을 드래그하여 움직이는 것도 가능하고 패널을 스와이프 해 지우기 또는 애니메이션 적용 등 다양한 기능을 활용할 수 있다고 한다.
AppBarLayout
LinearLayout을 상속한 스크롤 제스처에 몇 가지 동작을 할 수 있다.
이 레이아웃은 자식으로 ToolBar를 지니고 있다.
- 주의할 점은 AppBarLayout은 반드시 CoordinatorLayout의 바로 아래 자식이어야 한다고 한다
또한 스크롤을 인식하기 위해서는 형제로 선언된 뷰가 RecyclerView 같은 스크롤 가능한 뷰여야만 한다.
BottomNavigation
바텀 네비게이션도 확인할 겸 추가
바텀 네비게이션은 Android Navigation (네비게이션) / Jetpack - https://bumjae.tistory.com/26에서
사용하였기 때문에 설명 생략
dependencies {
implementation 'com.google.android.material:material:1.3.0'
}
build.gradle 머터리얼 디자인 추가
<style name="AppTheme" parent="Theme.MaterialComponents.Light.NoActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">#58FAAC</item>
<item name="colorPrimaryDark">@color/purple_700</item>
<item name="colorAccent">@color/teal_200</item>
<item name="windowActionBar">false</item>
<item name="windowNoTitle">true</item>
</style>
values / theme.xml 에 기본 툴바 대신 사용할 툴바의 스타일 지정 (기본 툴바 제거 설정 필요)
<?xml version="1.0" encoding="utf-8"?>
<layout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
tools:context=".coordinator.CoordinatorActivity">
<androidx.coordinatorlayout.widget.CoordinatorLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<FrameLayout
android:id="@+id/frame_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_behavior="@string/appbar_scrolling_view_behavior">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</FrameLayout>
<com.google.android.material.appbar.AppBarLayout
android:id="@+id/appbar"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<androidx.appcompat.widget.Toolbar
android:id="@+id/main_toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
android:theme="@style/AppTheme"
app:contentInsetStart="0dp"
app:layout_scrollFlags="scroll|enterAlways">
<ImageView
android:layout_width="?attr/actionBarSize"
android:layout_height="?attr/actionBarSize"
android:padding="5dp"
android:src="@drawable/ic_baseline_android_24" />
<androidx.appcompat.widget.AppCompatSpinner
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:entries="@array/ex_array" />
<androidx.appcompat.widget.SearchView
android:id="@+id/search"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</androidx.appcompat.widget.Toolbar>
</com.google.android.material.appbar.AppBarLayout>
<com.google.android.material.bottomnavigation.BottomNavigationView
android:id="@+id/bottom_navi"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="bottom"
android:layout_marginStart="0dp"
android:layout_marginEnd="0dp"
android:theme="@style/AppTheme"
app:itemBackground="@color/cardview_dark_background"
app:itemIconTint="?attr/colorPrimary"
app:itemTextColor="@color/white"
app:labelVisibilityMode="labeled"
app:layout_behavior="com.google.android.material.appbar.AppBarLayout$ScrollingViewBehavior"
app:menu="@menu/menu_bottom_navigation" />
</androidx.coordinatorlayout.widget.CoordinatorLayout>
</layout>
구성한 Activity의 Layout xml 코드이다.
binding 사용을 해서 layout으로 감싸줬지만 Coordinator만 사용해도 된다.
MainContent를 표시할 FrameLayout에 behavior 값을 적용해준다.
app:layout_behavior="@string/appbar_scrolling_view_behavior"
app:layout_scrollFlags="scroll|enterAlways"
툴바에서 app:layout_scrollFlags 속성을 이용하여 다음과 같이 스크롤 옵션을 지정할 수 있다.
- scroll: 스크롤 이벤트 적용
- snap: View가 부분적으로만 표시되면, View가 스냅 되어 가장 가까운 가장자리로 스크롤
- enterAlways: 아래로 스크롤할 때마다 이 View가 아래로 보임
- enterAlwaysCollapsed: enterAlways 속성과 비슷하지만 스크롤 뷰가 맨 위에 도달했을 때 전체 뷰가 보임
- exitUntilCollapsed: 스크롤을 아래, 위로 이동 시 View의 minHeight 만큼만 보이고 스크롤이 최상단에 도착 시 나머지 View의 전체가 보임
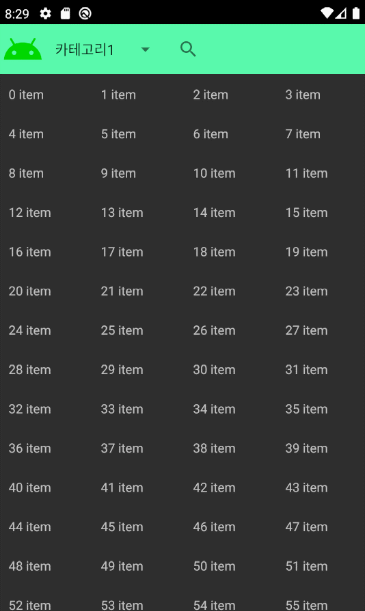
이렇게 구성 후 실행하여 보면 리사이클러뷰가 구성되어지고 스크롤을 해보면 상단바와 하단바가 숨김 표시 처리가 되는 것을 확인할 수 있다.
나중에 비슷한 기능 요구 사례가 있으면 참고해야겠다.
출처 : https://bumjae.tistory.com/31
Android Scroll 상, 하단바 숨기기 (CoordinatorLayout)
앱들을 사용하다 보면 스크롤할 때나 상단에 검색 툴바, 하단의 바가 사라질 때가 있고 나타날 때가 있다. 이러한 효과를 사용해보기 위함도 있고 코드본을 가지고 있기 위해 구현해 보았다. Coor
bumjae.tistory.com
Reference
https://readystory.tistory.com/127
'프로그래밍 > Android' 카테고리의 다른 글
[Android Studio] AVD 실행시 no longer exists 에러 (0) | 2023.05.15 |
---|---|
[Android] EditText 밑줄제거 및 커서 커스텀 (0) | 2023.04.24 |
[Android] TableLayout (0) | 2023.04.17 |
[Android] Dialog 중복생성 방지 (0) | 2023.04.14 |
[Android] 카카오 API 로그인시 302에러 (0) | 2023.03.30 |
댓글